What do you need to do in order for an app that can identify living objects using React? (r) (r)
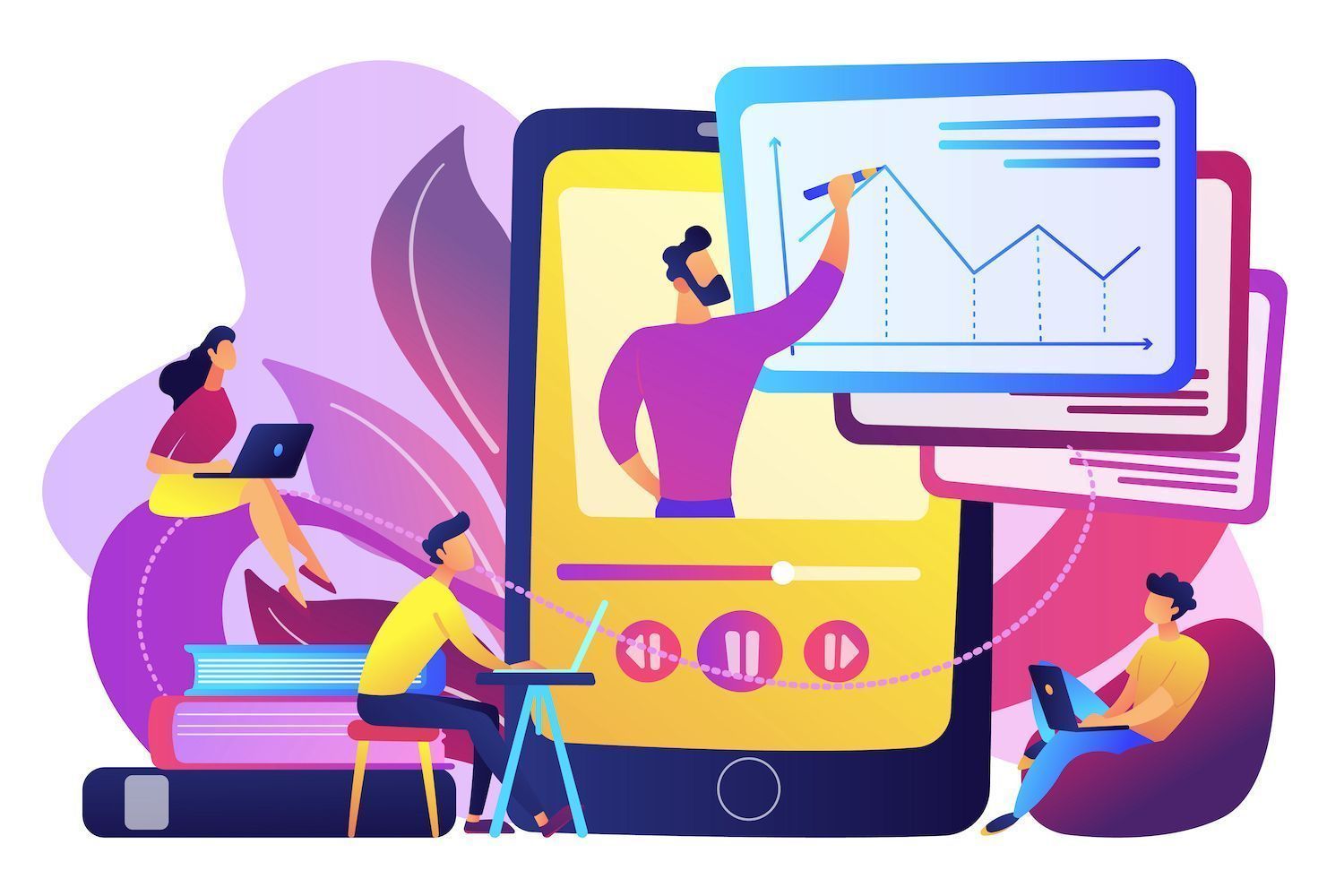
-sidebar-toc>
Cameras are getting more advanced and are becoming more sophisticated. real-time detection of objects is now an increasingly popular option. From autonomous cars, advanced surveillance systems, and even AR applications, this technology is used to a wide range of uses.
Computer Vision is the nebulous phrase used to describe a method that utilizes computers and cameras for different operations. It is, as mentioned previously, an extensive and intricate field. A lot of people don't think that they can start participating in the immediate searching for items using your browser.
The scenario
The following is a comprehensive list of the most important technologies used for this post:
- TensorFlow.js: TensorFlow.js is a JavaScript library that can bring the benefits of machine learning into your browser. It allows you to download previously developed models which have been trained to perform object recognition and run them through your browser. It removes the need to perform complex server-side processing.
- Coco SSD It is an object recognition system that is trained. It's known as Coco SSD which is a light model that has the capability of understanding the vast majority of objects commonly used. Even though Coco SSD is a powerful tool, it is important to be aware of the fact that it was created using a variety of diverse objects. If you're in need of a specific feature to detect, you can create a custom model with TensorFlow.js by this tutorial.
Create a brand new React project
- Make a brand fresh React project. This is easy to accomplish this using these guidelines:
NPM create vite@latest -object detection React template
This will generate the initial React project you are able to develop using the help of..
- Then, you'll be able install TensorFlow and the Coco SSD libraries using these commands within the project:
npm i @tensorflow-models/coco-ssd @tensorflow/tfjs
Now is the best time to develop your app.
Configuring the application
Before creating the code that creates the logic needed to recognize objects, take a take glance at the code which was written in this instructional. The user interface for the application might include:
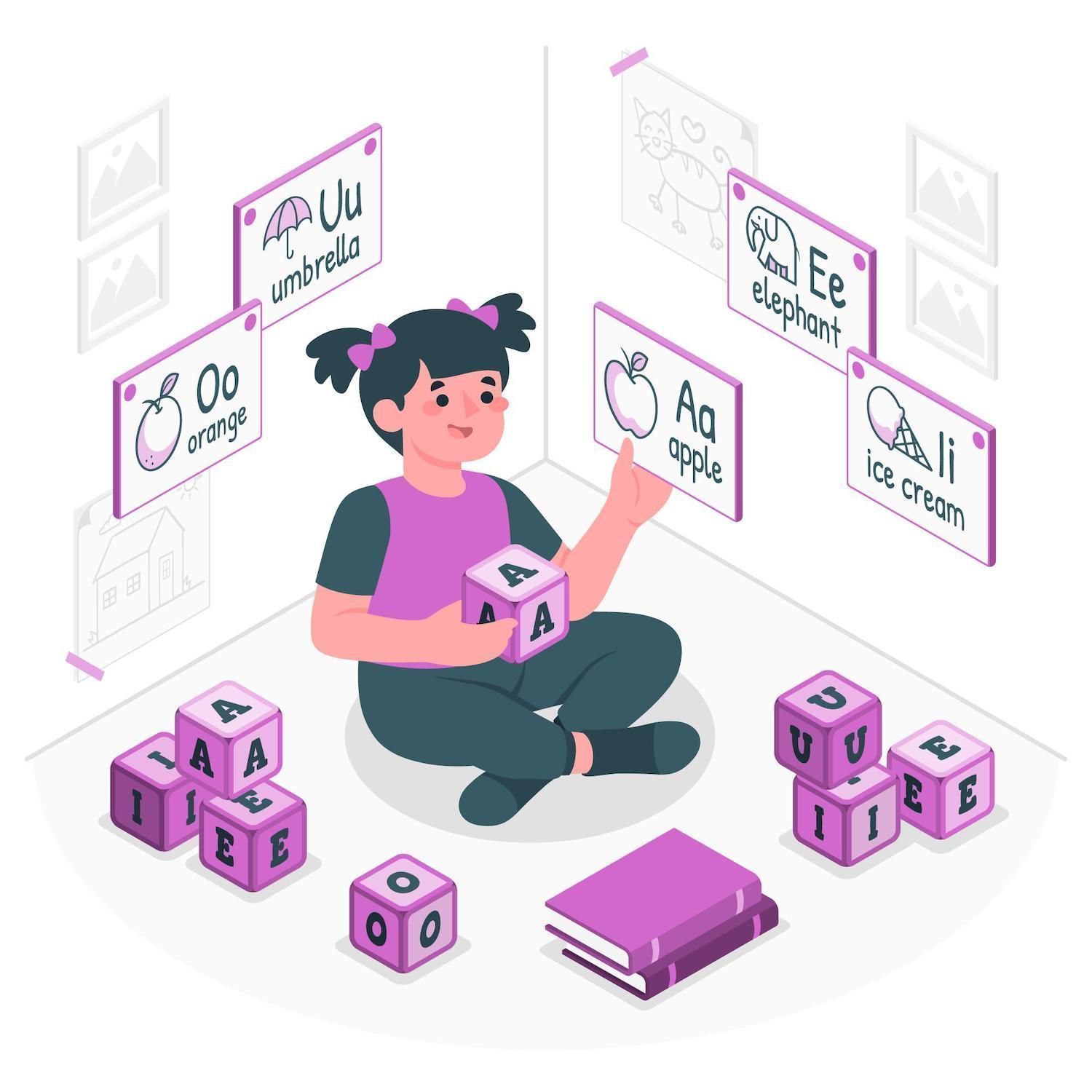
When users press the Start Webcam button after clicking the Start Webcam button, they're requested to authorize the app to utilize webcam feeds. When permission is granted, the app begins to show the live stream of the webcam. It can also identify objects it detects within the stream. Then, it creates an equilateral triangle to show what it sees within the feed and it labels the items. It then is able to label them.
The first thing to do is create a user-friendly user interface for your application. Copy these actions into app.jsx. App.jsx file:
import ObjectDetection from './ObjectDetection'function App() return ( Image Object Detection ); Export default App
The code fragment acts as the page's header. It also includes a customised component called "ObjectDetection"
. It will take the feed from a camera and locate objects on a moment's notice.
To build this component create a brand-new document titled ObjectDetection.jsx in your homedirectory and then copy the following code into it:
UseEffect and useState of'react'. Const objectDetection = () Const videoRef = useRef(null) Const [isWebcamStartedsetIsWebcamStarted], useState(false) Const setWebcam to be in sync () = /StopWebcam () > // TODO; return ( isWebcamStarted? "Stop" : "Start" Webcam isWebcamStarted ? : ); ; export default ObjectDetection;
This is how you could apply the code to build startWebcam. "startWebcam"
function:
const startWebcam = async () => try setIsWebcamStarted(true) const stream = await navigator.mediaDevices.getUserMedia( video: true ); if (videoRef.current) videoRef.current.srcObject = stream; catch (error) setIsWebcamStarted(false) console.error('Error accessing webcam:', error); ;
The system asks users to allow access to their webcam. Once granted, the system will change the webcam's video. video
will display the live feed of the webcam to any user who is able to access the site.
If the application is not able be connected to a feed of the camera (possibly due to the lack of a webcam in the device or another reason that the user wasn't granted the access) it will show an error message to the console. The console could display an error message which could explain the root of this issue to the user.
The next step is to substitute stopWebcam with the stopWebcam
function by using the following code:
const stopWebcam = () => const video = videoRef.current; if (video) const stream = video.srcObject; const tracks = stream.getTracks(); tracks.forEach((track) => track.stop(); ); video.srcObject = null; setPredictions([]) setIsWebcamStarted(false) ;
The code scans for videos which are available through the webcam
object and stop every one of them. After that, it will change the isWebcamStarted
state in the status to the true value.
.
In the same situation, it's possible to open the app to check if it's able to access and see the webcam feed.
The code must be pasted within your index.css file to make sure that the application appears exactly like the one you had earlier seen.
#root font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif; line-height: 1.5; font-weight: 400; color-scheme: light dark; color: rgba(255, 255, 255, 0.87); background-color: #242424; min-width: 100vw; min-height: 100vh; font-synthesis: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; a font-weight: 500; color: #646cff; text-decoration: inherit; a:hover color: #535bf2; body margin: 0; display: flex; place-items: center; min-width: 100vw; min-height: 100vh; h1 font-size: 3.2em; line-height: 1.1; button border-radius: 8px; border: 1px solid transparent; padding: 0.6em 1.2em; font-size: 1em; font-weight: 500; font-family: inherit; background-color: #1a1a1a; cursor: pointer; transition: border-color 0.25s; button:hover border-color: #646cff; button:focus, button:focus-visible outline: 4px auto -webkit-focus-ring-color; @media (prefers-color-scheme: light) :root color: #213547; background-color: #ffffff; a:hover color: #747bff; button background-color: #f9f9f9; .app width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: column; .object-detection width: 100%; display: flex; flex-direction: column; align-items: center; justify-content: center; .buttons width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: row; button margin: 2px; div margin: 4px;
The app.css file must be deleted. App.css file to make sure you do not ruin the design of your parts. You are now ready to implement the necessary technology of real-time object recognition in your app.
Implement real-time detection for objects
- Initial steps are to transfer the data into Tensorflow along with Coco SSD at the top of ObjectDetection.jsx :
import * as cocoSsd from '@tensorflow-models/coco-ssd'; import '@tensorflow/tfjs';
- Create a new condition in the
ObjectDetection
component, so as to preserve the prediction array generated by Coco SSD model: Coco SSD model:
const [predictions setPredictions, useStatesetPredictions, useState ([]);
- Then, you can create an application that is loaded into Coco SSD model. Coco SSD model. It loads into the Coco SSD model, loads onto Coco SSD model, collects footage and makes predictions:
const predictObject = async () => const model = await cocoSsd.load(); model.detect(videoRef.current).then((predictions) => setPredictions(predictions); ) .catch(err => console.error(err) ); ;
The program uses the video's feed to create predictions about objects that are within the feed. It provides you with an array of objects that are predicted to be visible. Each featuring a tag that includes a percentage of the levels of confidence and the numbers identify the position of the object inside the video's frame.
It is crucial to activate this feature to process videos in the order that frames are added. later use forecasts that are stored inside the forecasts
state. This will display boxes and labels of every known object in the video stream that is in live view.
- After that you'll be in position to utilize
the setInterval
function to call this function in a periodic interval. You must also ensure that the function is not active after the user is shut off from receiving feeds via their webcam. To prevent this happening, make use of yourClearInterval
feature within JavaScript.Add your state container as well as hooks for useEffect to theaffect
hooks in theelement, the detector of objects
element, in order to build anpredictObject
function. The program is running indefinitely while the webcam is active, however it will then taken off of the webcam when it's shut off.
const [detectionInterval, setDetectionInterval] = useState() useEffect(() => if (isWebcamStarted) setDetectionInterval(setInterval(predictObject, 500)) else if (detectionInterval) clearInterval(detectionInterval) setDetectionInterval(null) , [isWebcamStarted])
The application is designed to detect objects within the image of the camera every 500 milliseconds. It is possible to alter the number of milliseconds each second depending on how fast you'd like the speed of object detection, but you should be aware of the possibility of applying it frequently. This could lead to your application becoming a large portion of memory within the browser.
- When you've got the data to make your forecast, you're now able to utilize the data to develop a prediction. If you've got an estimate, it may be used to identify containers. The forecast can be used to display the label or even be used as a container on the live feed in the film. For this to happen, you'll need to alter to modify the
returning
declaration for yourlabels detection.
Enter the following data:
Return ( Is WebcamStarted ? "Stop" : "Start" Webcam isWebcamStarted ? : /* Add the tags below to show a label using the p element and a box using the div element */ predictions.length > 0 && ( predictions.map(prediction => return prediction.class + ' - with ' + Math.round(parseFloat(prediction.score) * 100) + '% confidence. ' > ) ) /* Add the tags below to show a list of predictions to user */ predictions.length > 0 && ( Predictions: predictions.map((prediction, index) => ( `$prediction.class ($(prediction.score * 100).toFixed(2)%)` )) ) );
The program shows the forecast list beneath the feed of the webcam. The program then creates an space around the object forecasted that includes coordinates for Coco SSD as well as names at the end of every box.
- for styling the boxes and labels correctly To style the labels and boxes correctly include this code in the index.css Index.css index.css file index.css file:
.feed position: relative; p position: absolute; padding: 5px; background-color: rgba(255, 111, 0, 0.85); color: #FFF; border: 1px dashed rgba(255, 255, 255, 0.7); z-index: 2; font-size: 12px; margin: 0; .marker background: rgba(0, 255, 0, 0.25); border: 1px dashed #fff; z-index: 1; position: absolute;
The application is complete. It is complete. The program has been capable of starting the server which developed it to test the program. What happens once the program has been completed
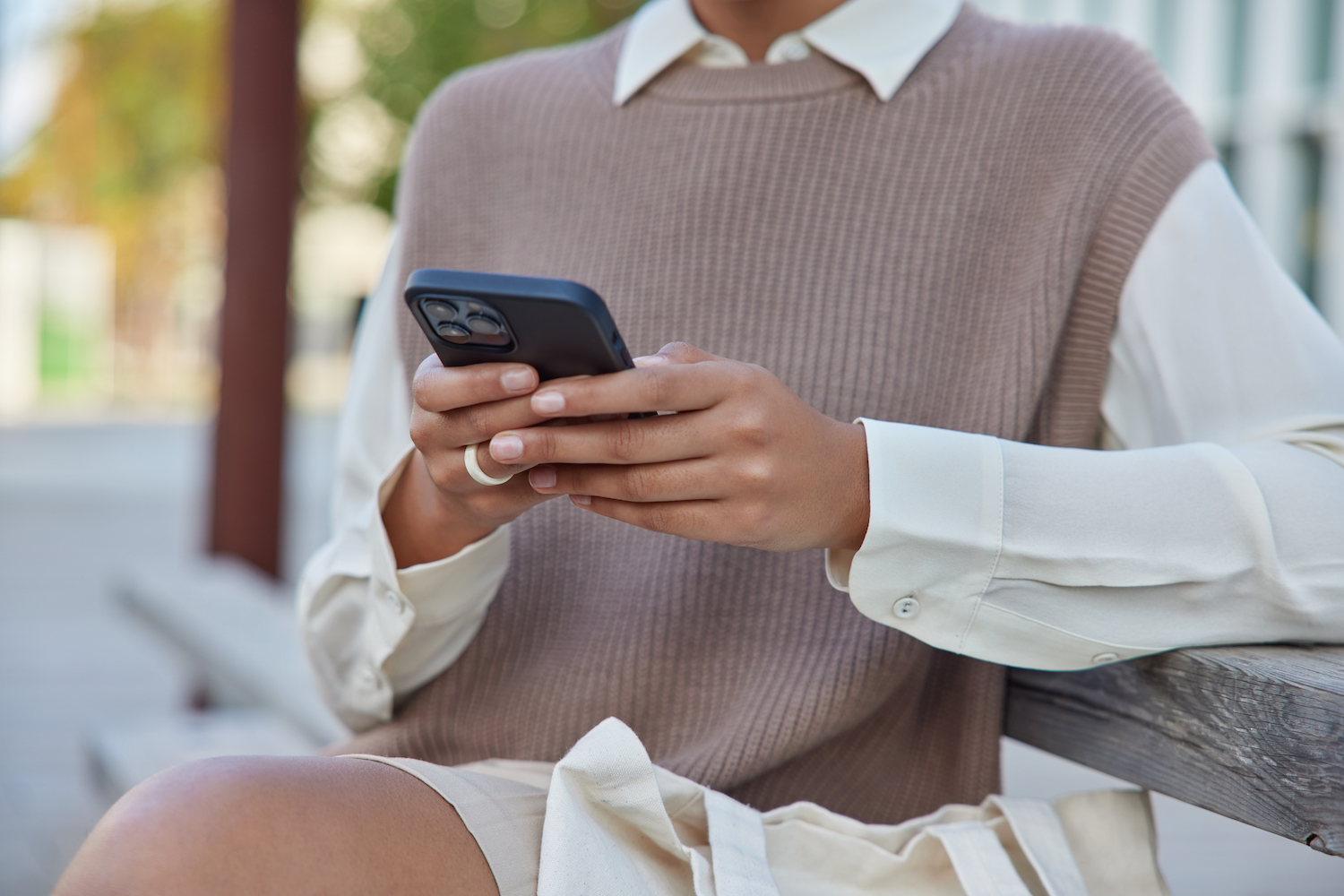
Complete code is available on the repository at GitHub. GitHub repository.
Install the application
If your repository on Git is up and running Follow these steps for installing the application :
- Create or sign in to an account to see the dashboard at Your Dashboard. My dashboard.
- Authorize your Git service provider.
- Select those static websites on the sidebar to left. Select Add Site. Choose to Add Website.
- Pick the branch you want to access and then the repository you wish to gain access through.
- Assign a unique name to your site.
- Integrate the setting for building according to the following format:
- Command to build:
yarn build
orNPM build
- Node version:
20.2.0
- Publish directory:
dist
- Then, click Create site.
After the app has been created, once the app has been launched, you can click "Visit the site" from the dashboard to start the app. It can be tested on different cameras of various devices to see how it performs.
Summary
It's had great success in the creation of the object recognition technology that is real-time in addition to live-time applications that is made using React, TensorFlow.js, and . This lets you explore the possibilities of computer vision as well as build interactive experiences using your browser.
Be aware that the Coco SSD model we used could only serve as a foundation. If you'd like to continue investigating the options, look into the possibility of being able to alter the method of detecting objects using TensorFlow.js which allows you to customize the app to identify those objects which best satisfy the particular requirements of your organization.
There is no limit to what you can do! This app could be the foundation for creating advanced applications like Augmented Reality Experiences, as well as innovative surveillance tools. If you are able to launch your app via the safe platform, you are able to make your app available to everyone around the world and watch the potentialities of computer vision come into the forefront.
What's the most difficult issue you've encountered which you think the real-time detection of objects could solve? Tell us about your experiences in the section of comments below!
Kumar Harsh
Kumar is an author of technical software and also an editor with his home in India. He's an expert on JavaScript and DevOps. Find out more on his expertise by visiting his site.
The original article was published this website.
The article was published on here
The post was published on this site.
This post was posted on here