Master TypeScript development in Visual Studio Code - (r)
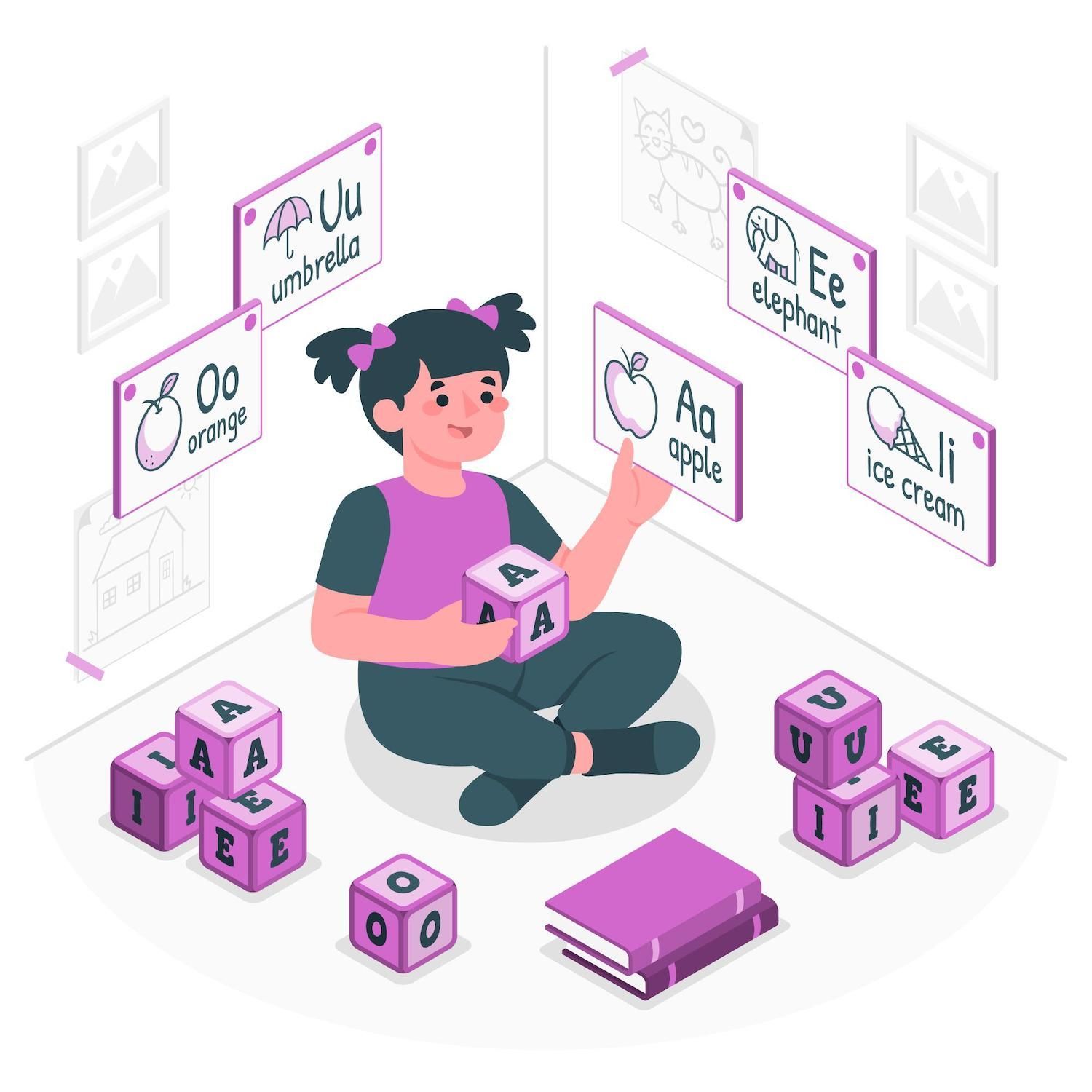
-sidebar-toc>
What do you need to set up Visual Studio Code for TypeScript development?
This step-by-step tutorial shows how to set up Visual Studio Code for TypeScript development. The first step is to create a Node.js project with TypeScript write some code, and execute, compile and then analyze the TypeScript then run it through Visual Studio Code.
The requirements
Before beginning, make sure you have:
- Node.js installed and localized
- Visual Studio Code was installed and downloaded
node-v
This will give you the most recent version of Node.js on your machine as follows:
v21.6.1
We're ready to get started on TypeScript by using Visual Studio Code!
Install the TypeScript compiler
Visual Studio Code supports TypeScript development, but it doesn't have the TypeScript compiler. Since the TypeScript compiler transforms or converts- TypeScript code to JavaScript and reverses the process It's a must for testing the performance of your TypeScript code. Also, tsc is a program that takes TypeScript Code as an input and produces JavaScript code to output following that you can use the JavaScript code with Node.js and it's Web browser.
Enter the below command into your terminal to install the TypeScript compiler globally on your personal computer:
NPM install Typescript
Make sure you are using the latest version in use of TSC:
tsc --version
If the command doesn't produce an error message, it is using tsc. This gives you everything you require to start building a TypeScript project!
Make an TypeScript project
Let's make a basic Node.js TypeScript project called hello-world. Open your terminal, and create a folder in which to keep the project you've created:
mkdir hello-world cd hello-world
Inside hello-world you can start your project with the following npm command
NPM init with a y
It creates it's package.json config file that you will need for the Node.js project. It is time to look at what the project consists of in Visual Studio Code!
Launch Visual Studio Code and select the File > Open Folder...
Then, in the pop-up window that comes up, click on the hello-world project folder and then select "Open". The project will look like the following:
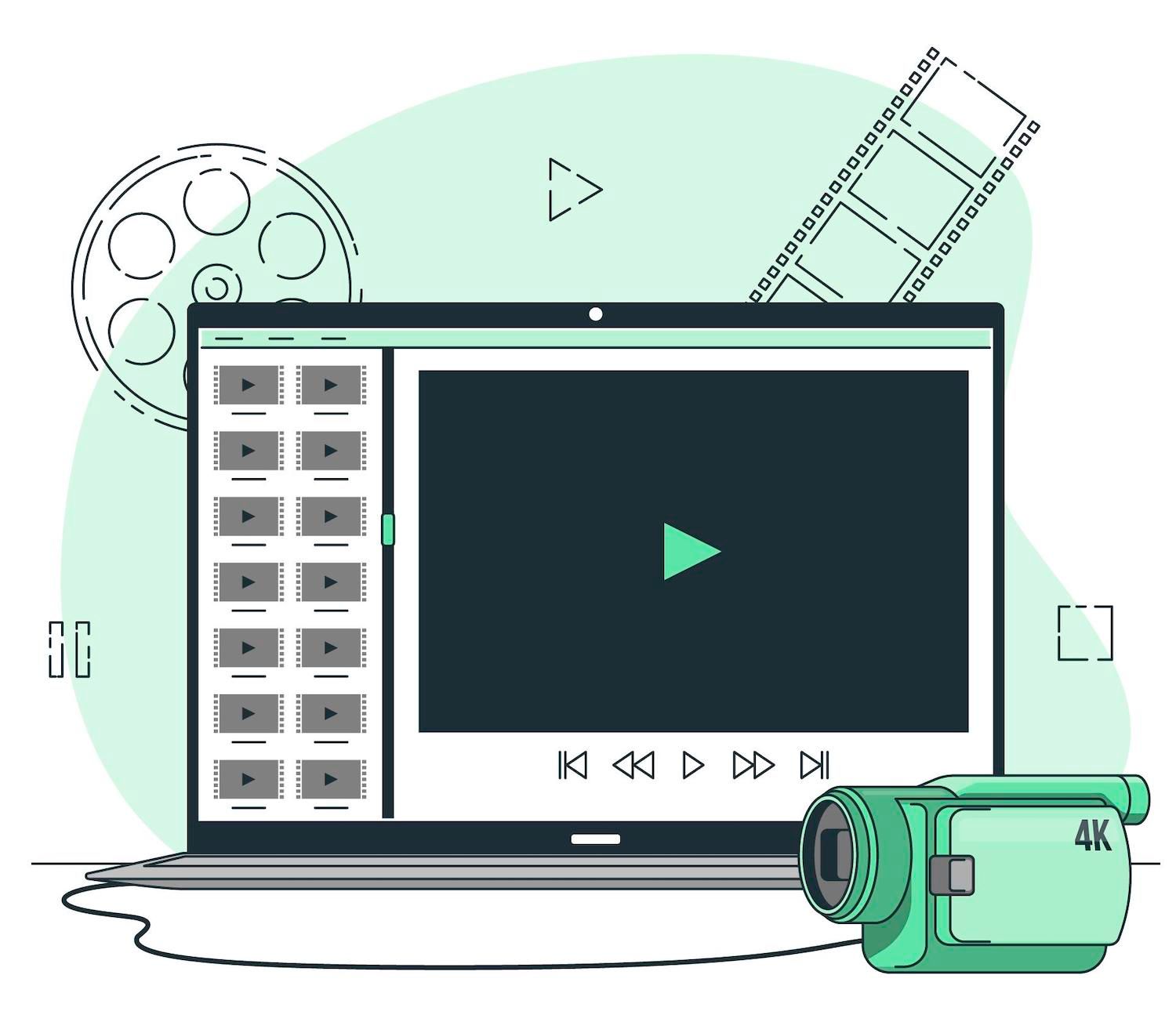
Currently, the project consists of an initial package.json file initialized by the init function used by npm
.
Choose from the menu View > Terminal option from the Visual Studio Code menu to gain access to Visual Studio Code's built-in terminal. Next, execute the following commands:
Npx TSC --init
It opens by creating a TypeScript configuration file called tsconfig.json located in the directory of the project.
The tsconfig.json file allows you to modify the behaviour of the TypeScript compiler. It also provides the TypeScript compiler, along with the instructions to switch it over to TypeScript code. Without it, tsc won't have the ability to develop your Typescript project in the manner you'd prefer.
You can open tsconfig.json in Visual Studio Code in Visual Studio Code, and look for comments on every setting. We want our tsconfig.json file to contain the following options:
"compilerOptions": "target": "es2016", "module": "commonjs", "esModuleInterop": true, "forceConsistentCasingInFileNames": true, "strict": true, "skipLibCheck": true, "sourceMap": true, "outDir": "./build"
It's likely that the one distinction you'll see between these options is the settings of the source mapping for the JavaScript produced by the software as well as the inclusion of an output directory.
"sourceMap": true, "outDir": "./build"
Modify the tsconfig.json file.
Maps to Source is an essential feature for Visual Studio Code compiler. Visual Studio Code compiler.
OutDir configuration. This OutDir configuration defines the location where the compiler will place the converted files. By default, that's the main folder for the project. To avoid cluttering the folder of your project with build files at every new creation, you could change the location of the folder similar to it's build directory..
The TypeScript project is now completed and ready to be built. First, however, you need to possess TypeScript codes.
Right-click in the Explorer section, then choose Create File... Type index.ts
and press Enter. Your project should now include the TypeScript document called index.ts:
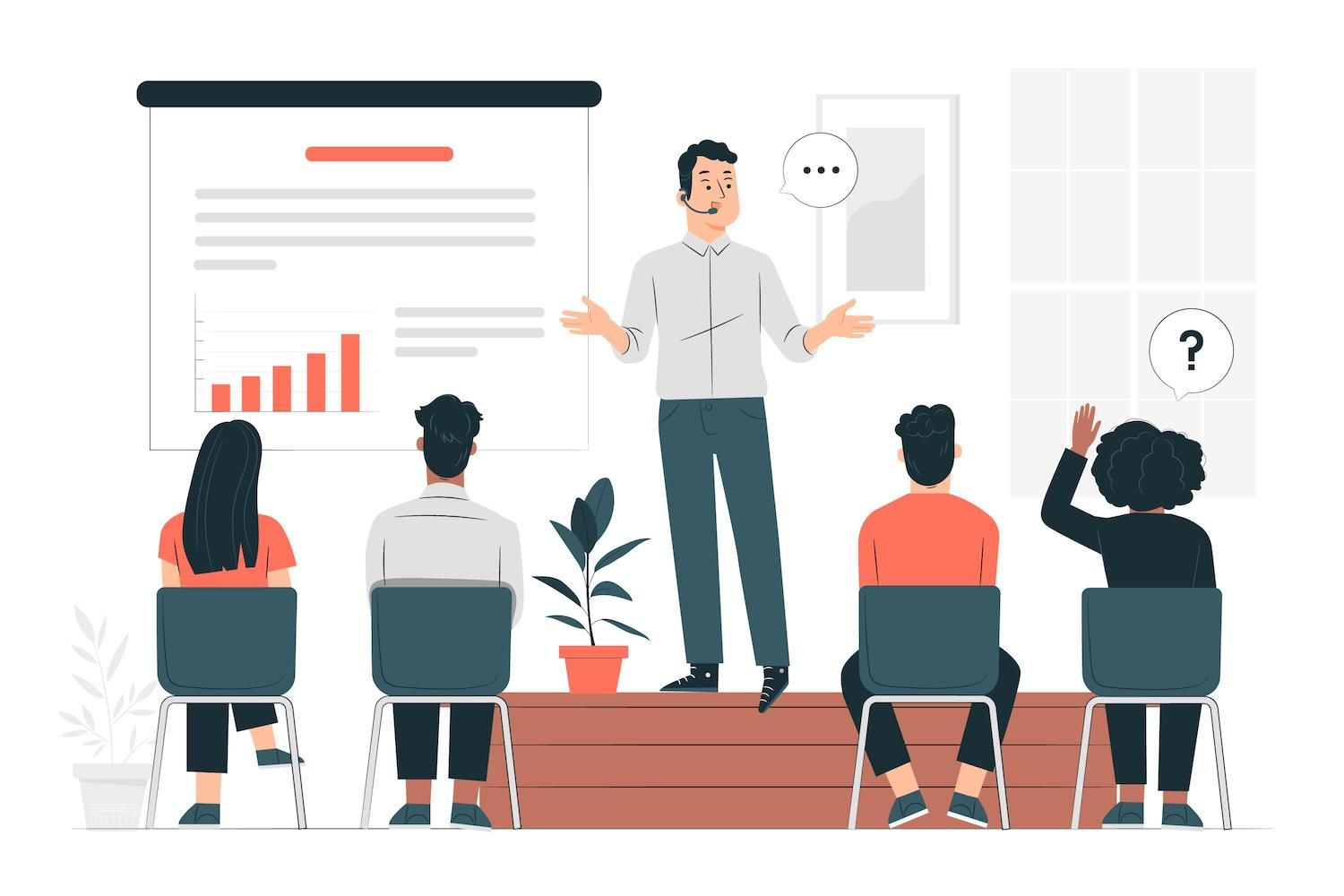
Let's begin by using the following TypeScript code:
const message: string = "Hello, World!" console.log(message)
This code fragment prints the famous "Hello, World! message.
Try IntelliSense for code completion
While writing the lines above in Visual Studio Code, you may have seen some code suggested by editors. The reason for this is IntelliSense the most popular the Visual Studio Code's powerful tools.
IntelliSense offers capabilities like the completeness of your codes, information about docs, and parameter info on functions. IntelliSense offers suggestions on how you can complete the code as you type. This can dramatically improve your productivity as well as accuracy. You can see it in action here:
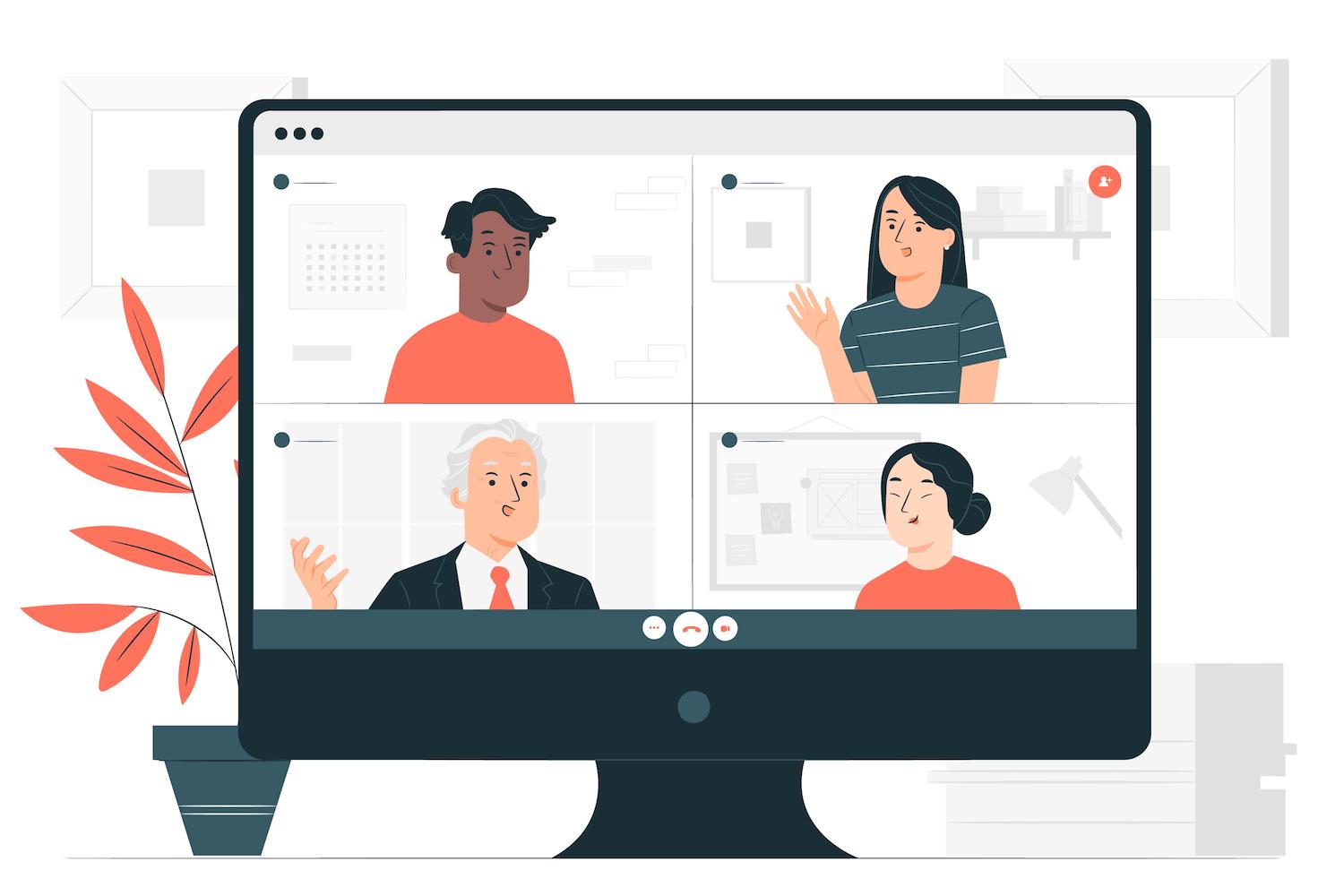
Remember the case that Visual Studio Code comes with IntelliSense support for TypeScript applications from the beginning. It is not necessary to set up the feature manually.
If you've learned how write TypeScript effortlessly in Visual Studio Code, let's compile it and see whether it's working.
Compiling TypeScript in Visual Studio Code
Open the terminal that is integrated into Visual Studio Code and run:
tsc -p .
This converts every TypeScript document within the project into JavaScript. The "-p .
tells that the compiler to use the tsconfig.json file located within the directory of current. In this case, the output, index.js and index.js.map as well as the original map index.js.map -- is located in the ./build directory.
You can confirm that the transposed JavaScript code is working when you use this command on the terminal.
Node ./build/index.js
Node.js will translate index.js and print on the terminal:
Hello, World!
An alternative method of starting the transpiler can be done by choosing the Terminal > Launch Building Task... on the Visual Studio Code menu and select the build tsc - tsconfig.json option.
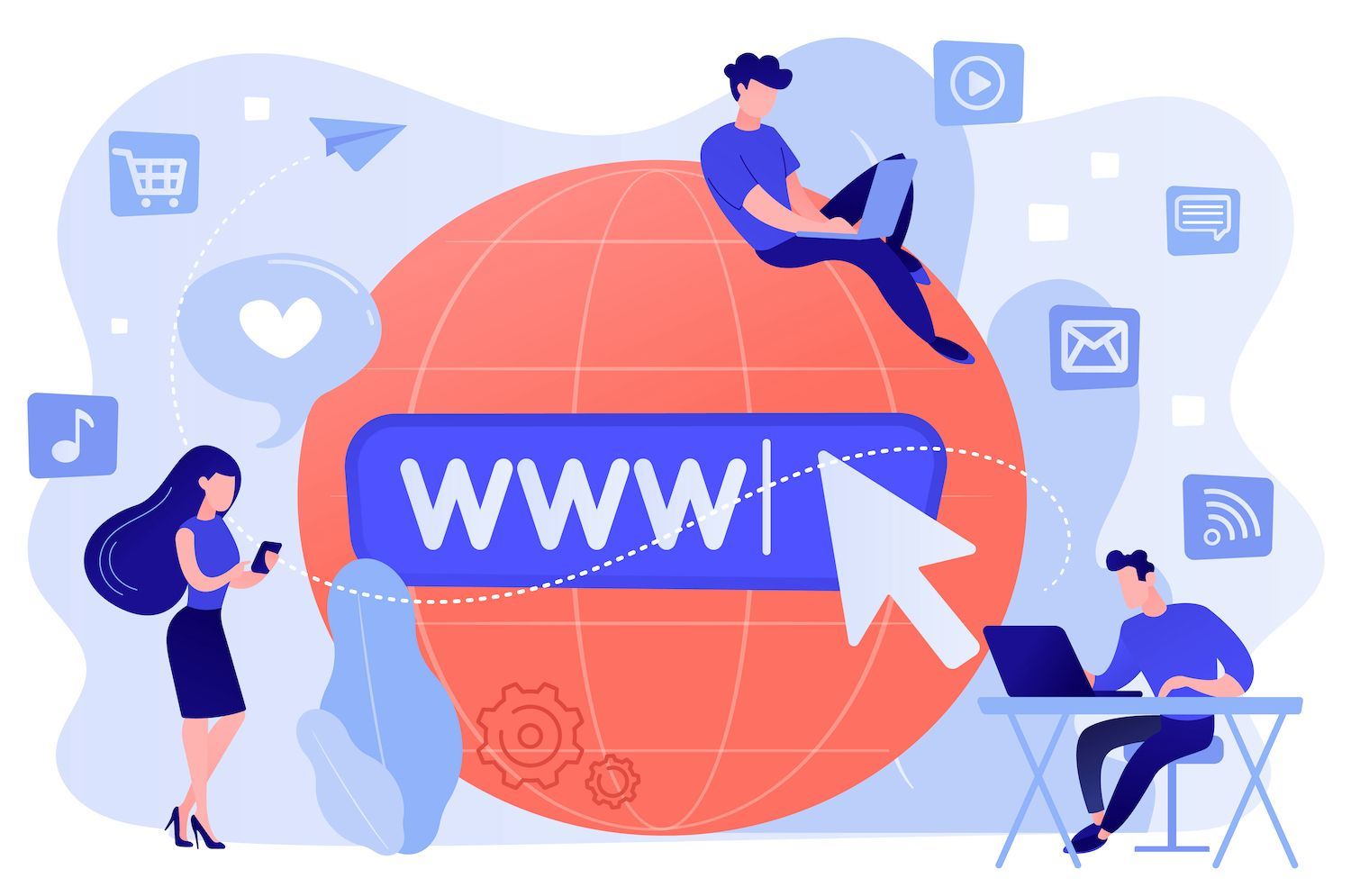
This command executes tsc -p .
behind the scenes and then builds your code in the editor.
It's the way to build your TypeScript project by using Visual Studio Code. It is now time to find out the steps to start and then debug your Code.
Run and debug TypeScript within Visual Studio Code
Visual Studio Code allows TypeScript debugging with its integrated Node.js debugger. Before you're able to utilize it, you'll have to make the file. Click the run and debug icon that is located on the left sidebar, click Create an launch.json file after which click Node.js.
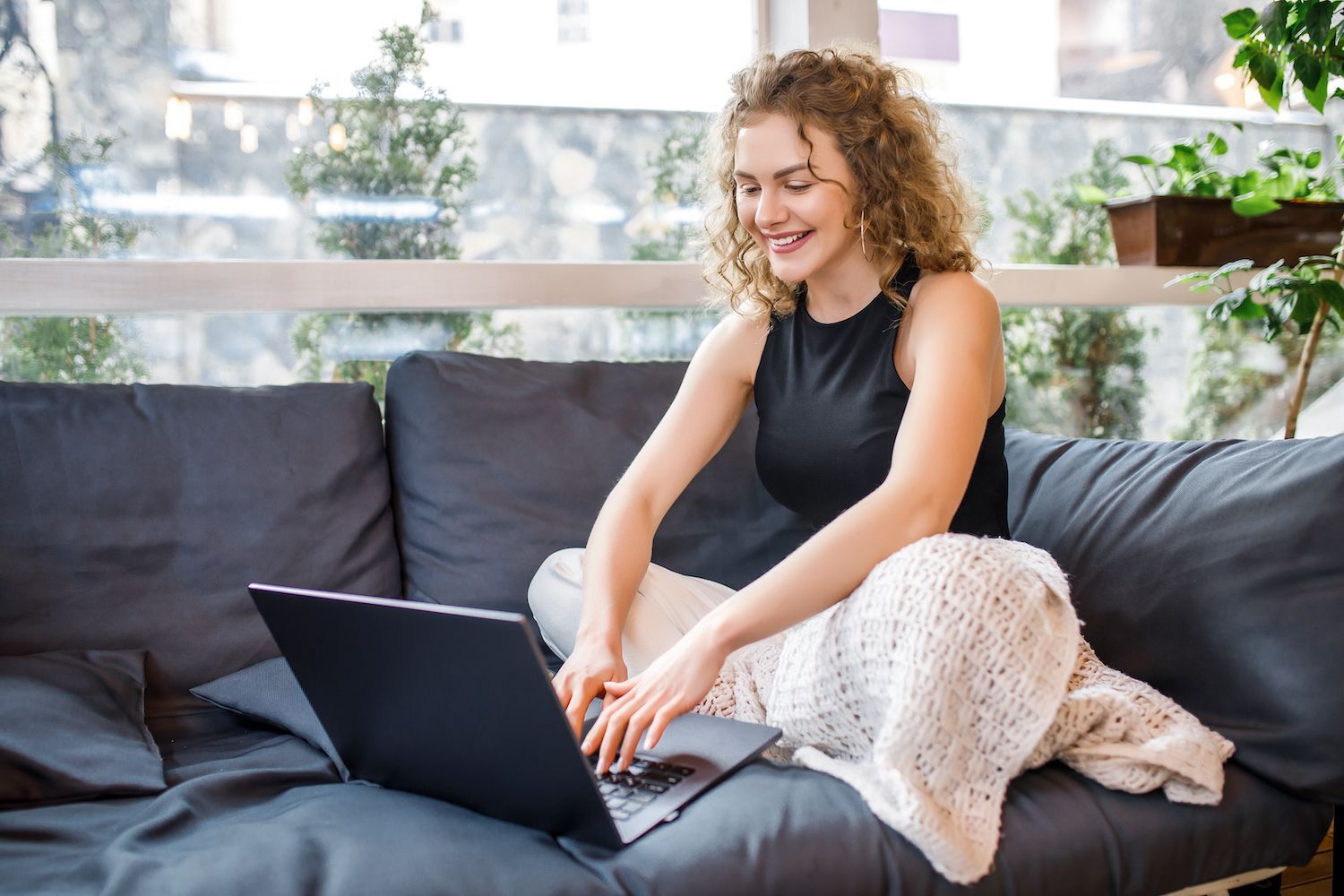
The process creates a new Node.js launch.json file which contains the configuration files that Visual Studio Code Visual Studio Code Debugger utilizes for debugging as well as launching applications. The configuration file outlines what the program will run as well as the command line arguments that it will use, and the environment variables which need to be defined.
Within the Explorer section, launch.json is located in the .vscode folder of the application.
Make changes as follows:
// Use IntelliSense to discover possible attributes. You can view the explanations of attributes currently in use. // For more information, visit: https://go.microsoft.com/fwlink/?linkid=830387 "version": "0.2.0", "configurations": [ "type": "node", "request": "launch", "name": "Launch Program", "skipFiles": [ "node_modules/**" ], "program": "$workspaceFolder/index.ts", "preLaunchTask": "tsc: build - tsconfig.json", "outFiles": ["$workspaceFolder/build/**/*.js"] ]
Make adjustments to your programs
, preLaunchTask
outFiles and preLaunchTask outFiles
choices, when you consider that
program
: Specifies the path that leads to the entry point of the application to debug. If using TypeScript it has to contain the primary file, which is executed at the start of the application.PreLaunchTask
is the codename for the Visual Studio Code build task which is run prior to launching the program. In the context of a TypeScript Project, this would be the job to create.outFiles
contains the path for the converted JavaScript files that are generated during the building process. The source map files created through tsc thanks to"sourceMap", an"sourceMap": true
config are used by debuggers to translate your TypeScript source code to resulting JavaScript code. This lets you debug TypeScript code in real-time.
Make sure to save your launch.json file and open index.ts. Choose the blank line prior to the console.log()
line in order to establish an endpoint. A red dot appears next to the line. It appears as:
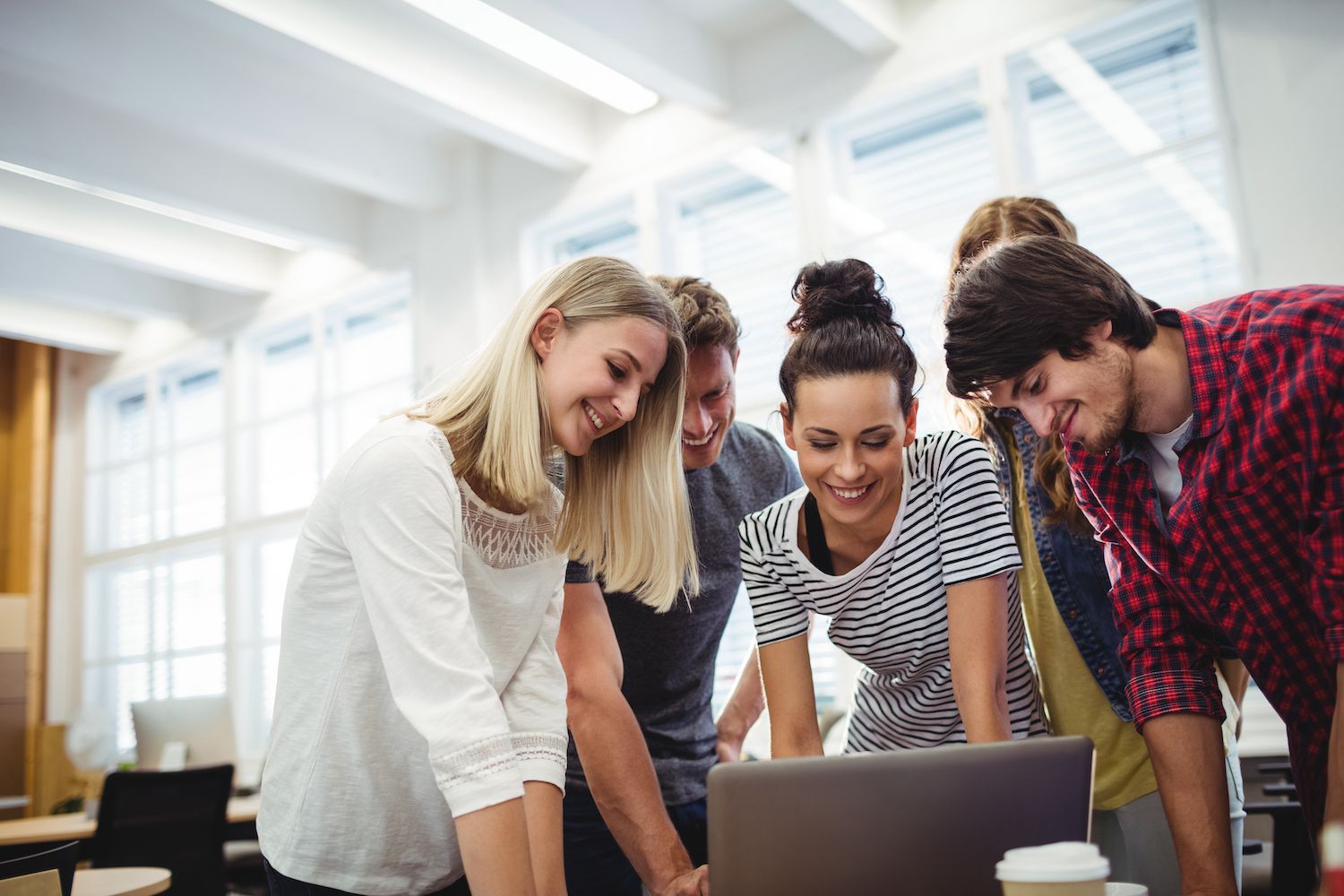
If you execute your code using the compiler, it's program is run but ceases at that point. With this breakpoint it is feasible to determine if the Node.js debugger that is available in Visual Studio Code is working exactly as you expect it to.
You can go to the Debuggle and Run section and then click the green play button. This will start the debugger. Wait until preLaunchTask
to run. When the code is completed the program will begin to run alongside the program. After that, it ceases execution at the breakpoint set in.
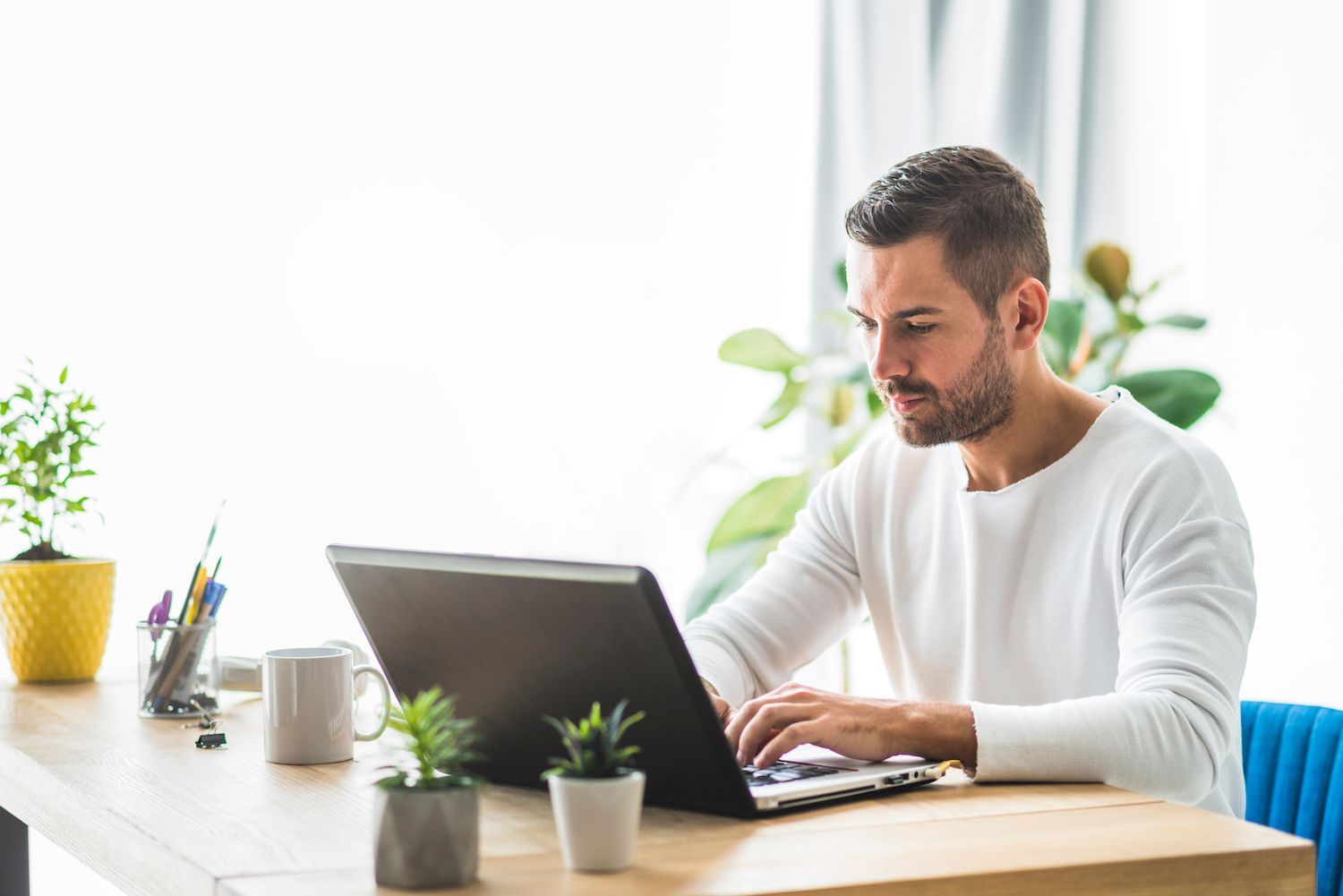
On the left in the above image, it's an option to examine the current values of variables as of the break. Also, you can stop, step over the break, move in or out as well as restarting and stopping, as described in the Visual Studio Code guide to the debugging process.
Make use of F5 to restart the process. You should then be able see the following message on the Debug Console tab:
Hello, World!
This is the kind of output you'd expect this application to produce. This is a sign that the program has been successfully executed.
It's now time to configure Visual Studio Code for TypeScript programming. This guide may be over, but there's one more essential thing you need to understand: how best to configure an extension within Visual Studio Code that can allow you to write high-quality code in TypeScript much easier.
What do I need to do to configure ESLint in Visual Studio Code
The most eagerly awaited Visual Studio Code extensions for TypeScript development is the ESLint extension.
ESLint is a well-known static code analysis tool for JavaScript and TypeScript that helps developers identify and correct common errors in coding and to ensure that the coding standard is strictly adhered to. This extension is able to run ESLint directly within the editor.
Let's incorporate ESLint with Visual Studio Code in your TypeScript project.
First, start by connecting ESLint for your project using the terminal command:
NPM init @eslint/config
During the configuration process, you will be asked some questions which will help you when creating the ESLint Configuration File. Your answers could be as follows:
What would you prefer using ESLint? * Style What kind of modules is your project using? Commonjs What framework do you use for your project? * none Does your project use TypeScript? Do you know where your code be being executed? BrowserHow do you want to establish an aesthetic for your project? * GuideWhat style guidelines do you like to follow? * standard-with-typescript What format do you want your config file to be in? * JSON
The program will look for dependencies and ask for permission to install any of the applications that are not yet already installed. You can respond like this:
Would you like to install them right now? If yes, which is the package manager you would want to set up? * NPM
After you've finished the procedure, you'll find the new .eslintrc.json file containing the above code in its first form:
"env": "browser": true, "commonjs": true, "es2021": true , "extends": "standard-with-typescript", "overrides": [ ], "parserOptions": "ecmaVersion": "latest" , "rules":
The .eslintrc.json file contains the settings used by ESLint to guarantee specific code design and quality standards. The standard .eslintrc.json for a Node.js TypeScript project may look as follows:
"env": "browser": true, "commonjs": true, "es2021": true, // enable node support "node": true , "extends": "standard-with-typescript", "overrides": [ ], "parserOptions": "ecmaVersion": "latest", "project": "tsconfig.json" , "rules": // force the code to be indented with 2 spaces "indent": ["error", 2], // mark extra spaces as errors "no-multi-spaces": ["error"]
The time has come to install the ESLint extension for Visual Studio Code. Click on the Extensions icon on the menu on the left side Then search for "ESLint" into the search field
. Locate the ESLint extension, then select the option to download it..
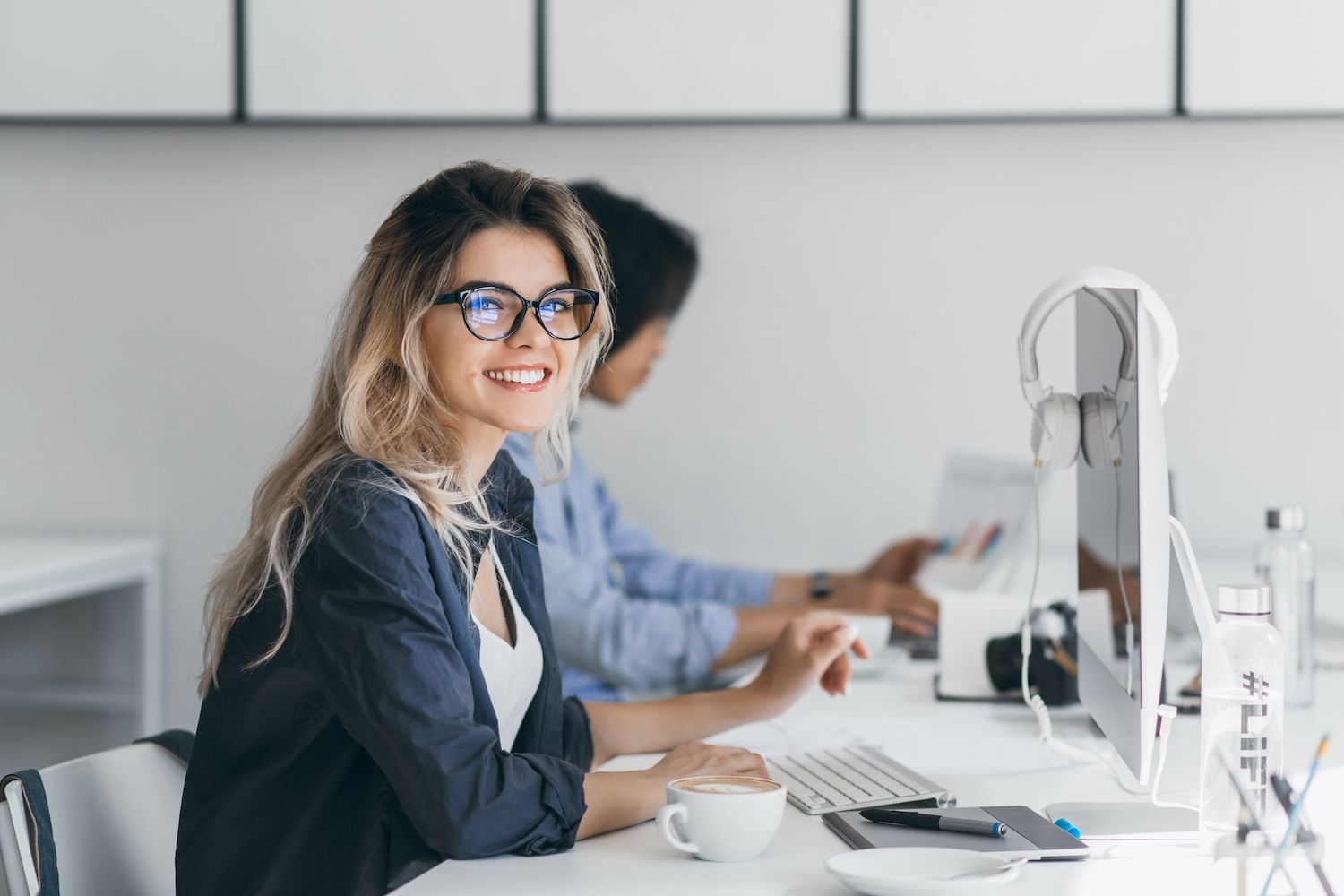
If you want to activate the ESLint extension to instantly verify your TypeScript files each time you save, create a settings.json file inside .vscode with the below content:
"editor.codeActionsOnSave": "source.fixAll.eslint": true , "eslint.validate": [ "typescript" ], "eslint.codeActionsOnSave.rules": null
Settings.json The settings.json file contains the configuration used by Visual Studio Code to customize how the editor behaves and the extensions they come with.
Start Visual Studio Code to make it run the most recent extension and settings.
If you go to index.ts and edit the code, there will be new errors reported in the IDE. For fixing code style errors, it's suggested that you save your file. ESLint immediately reformats the code as defined in .eslintrc.json.
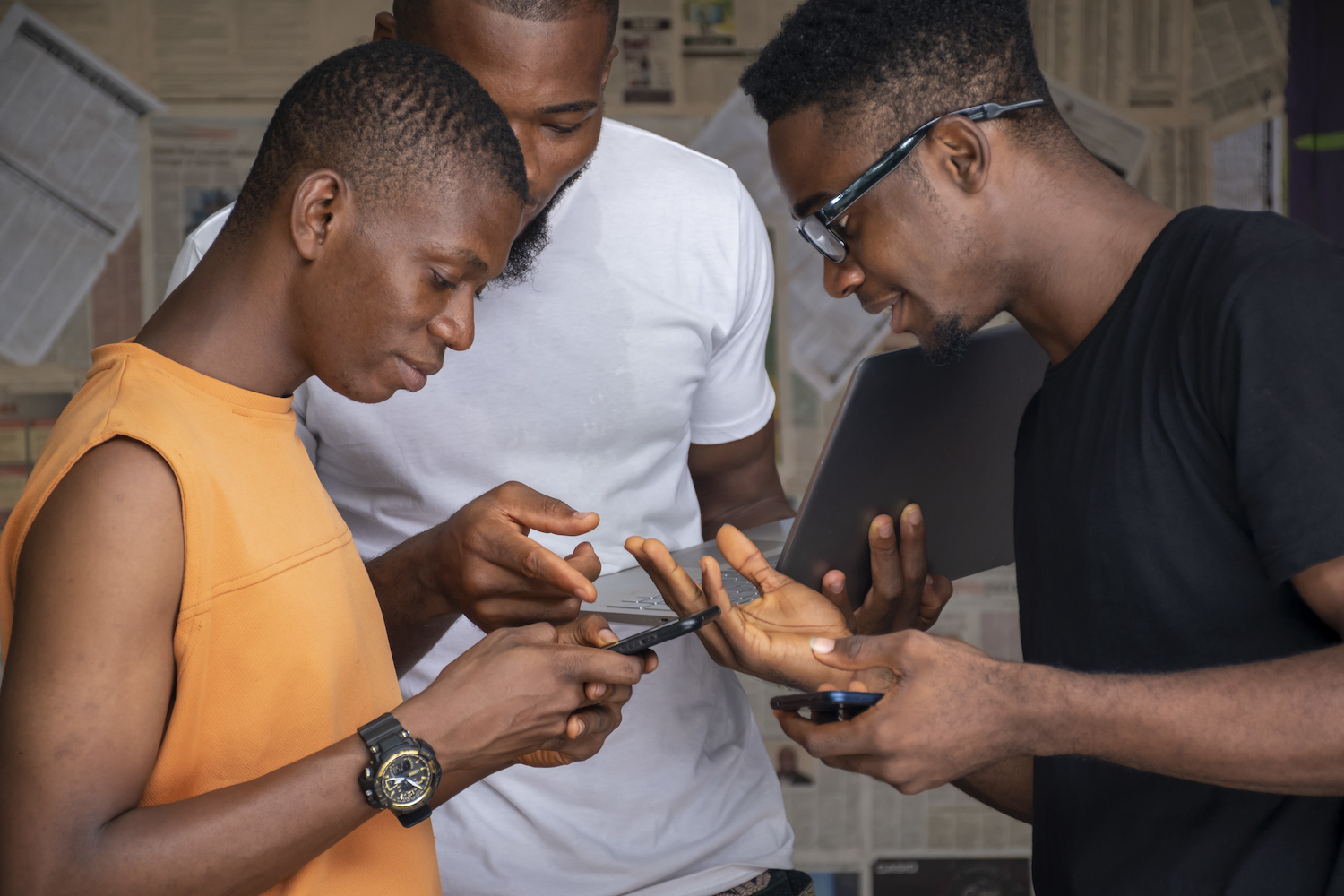
It's now possible to stop the development of high-quality code! The only thing left to complete is to connect your Node.js application to a reputable cloud hosting platform like's.
Summary
So, setting up Visual Studio Code for development by using TypeScript is quite simple. You have just discovered how to build the Node.js project in TypeScript after which you load the project into Visual Studio Code, and make use of the IDE to write code assisted by IntelliSense. You also set up to use the TypeScript compiler, installed the Node.js compiler to debug TypeScript programming, and integrated ESLint in the project.
Antonello Zanini
Antonello is an engineer in software, but prefers to be known as a Technology Bishop. Writing about knowledge is his primary goal in his job.
This post was posted on here