Laravel Eloquent Relations User's Guide for Advanced user (r) (r)
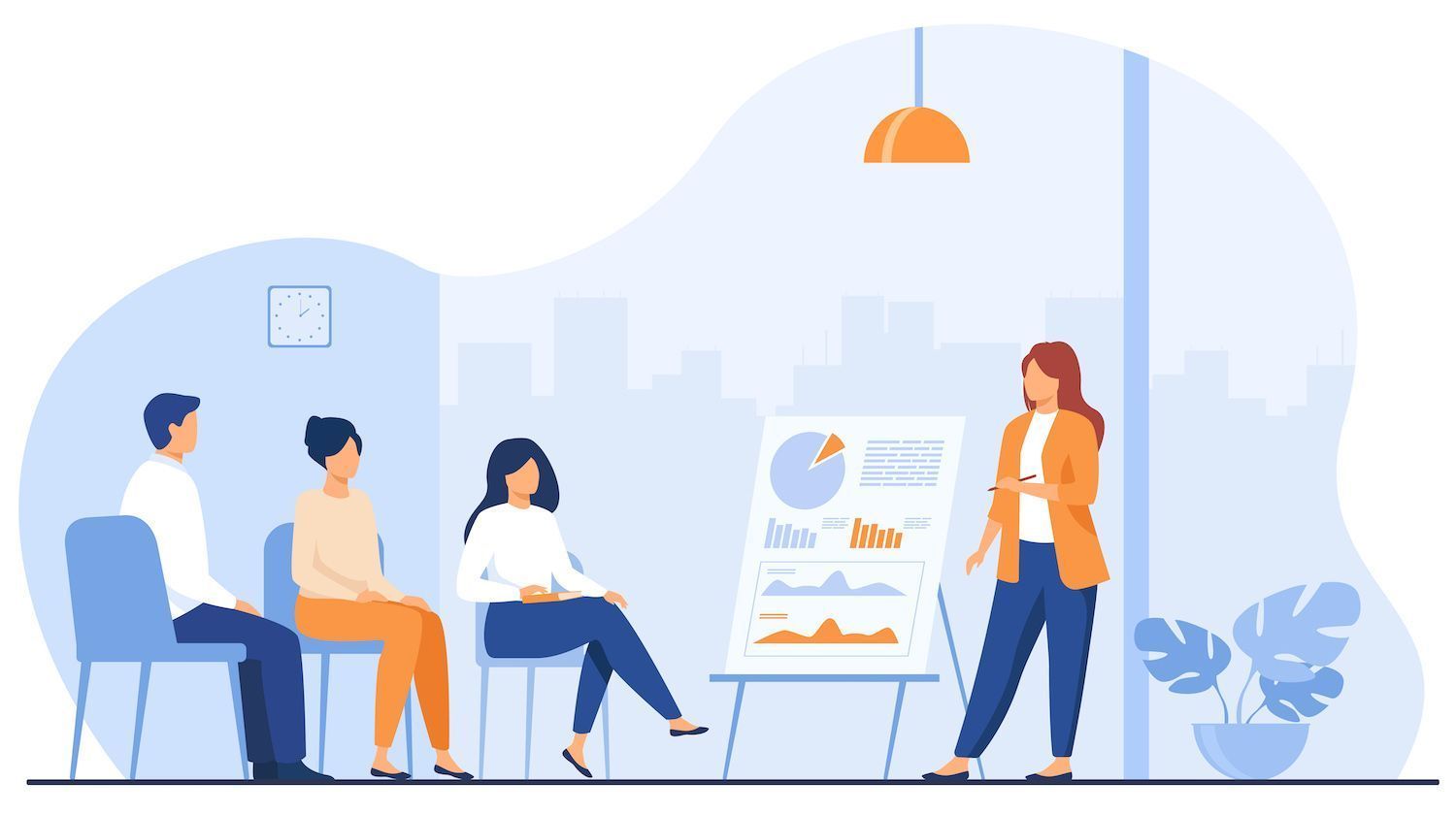
Please share the information with
There is a time and a possibility for any programmer to connect to databases. This is the reason that Eloquent has been transformed the Laravel ORM (ORM) permits you to communicate via tables in databases. It's simple and fast.
Experts are aware and understand the six most crucial types of relationships that need to be analyzed and discussed.
What is the relationship between Eloquentians to their own language?
In working with tables in the relational database, you'll be able recognize connections via the hyperlinks between tables. This lets you manage the information and organize effectively, which improves the readability and handling the information. There are three types of databases that are used to keep real-time information
- one-to-one: A single record from the table could be connected to a different one, but there is only one table. For example, a name, or Social Security number.
- One-to-many can be linked to multiple records from other tables. Similar to a writer's blog and.
- Tables with multiple entries are linked to several records in a different table. This includes students and their courses they're in.
Laravel provides a straightforward method to handle connections and manage them to databases with an object-oriented syntax in Eloquent.
In addition to the definitions above, Laravel also adds other connections, which include:
- There's a myriad of methods to explore
- Polymorphic Relationships
- Polymorphic
Take, for example, an online store that contains many different items, that all fall into the same category. Therefore, splitting databases into different tables is logical from a practical point of view. Every table is subject to particular challenges that are particular to it, and it may not be necessary to go through every table.
There is a way to create an initial one-to-many relationship with Laravel for us to assist in times when you'd like to find out more information about the products. This can be done through the model of Product.
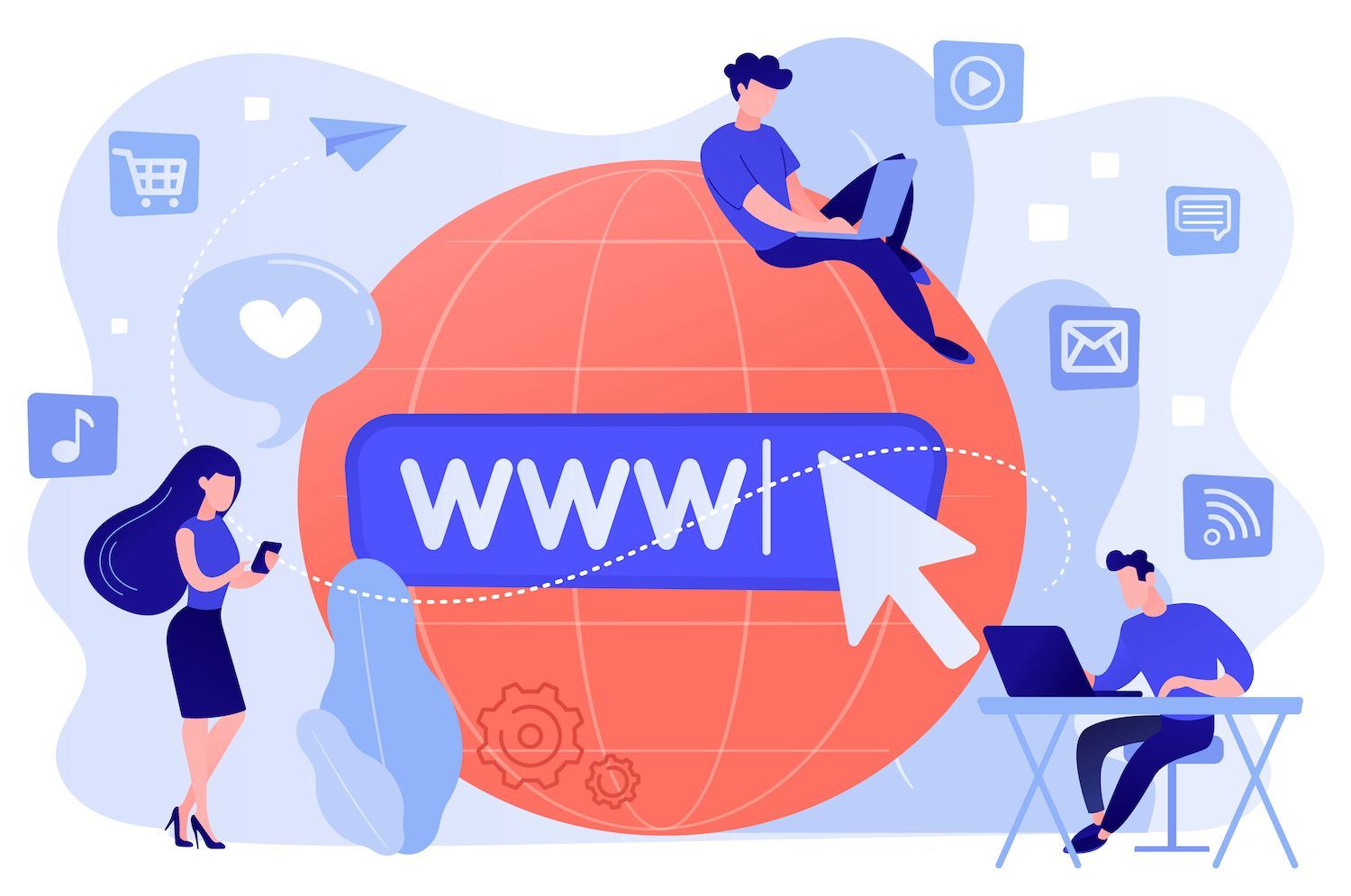
One-to-One Relationship
In the first connection Laravel offers, they join two tables in a way to ensure that the row of the table is connected to a single row, however only one table.
In order to gain more understanding on the subject, it is necessary to design two models that contain every motion:
php artisan make:model Tenant Php artisan make:model Rent
The situation is a mix of two kinds. One is that of the tenant with the rent.
hasOne(Rent::class);
It's because it is deciding to establish a foreign key relation with its parent name (Tenant in this case) and Rent models are based on the idea that there exists an ID number associated with the tenant that is an external key.
The method can be modified in using this method, for example, by adding arguments for the following method method: isOne method
Return"$this" >hasOne(Rent::class, "custom_key");
Eloquent will presume that the key is in fact an association with the external key specified by the primary key of its parent (Tenant Model). It will by default look at the tenant's ID who is associated with keys ID keys that are associated with the ID key in the Tenant Model record. It is possible to overwrite the ID of the tenant by making use of an alternative argument inside the isOne. is one method. It's in line with another vital
return $this->hasOne(Rent::class, "custom_key", "other_key");
If we've identified the one to 1 relationship between the models and models, it's possible to apply the relationship to make it simpler such as:
$rent = Tenant::find(10)->rent;
This specific line determines the rent for the tenant based on the 10th number if there is the ID is present.
One-to-Many Relationship
The same manner as the relation that was previously mentioned, this will make it clear that there is a link between single parent models aswell when models have multiple children. There is a good chance that the tenant isn't paying one rent installment since the installment is normal. Therefore, it's most likely that there will be a series of installments.
The previous relationship was not ideal, and is one that we're capable of fixing:
hasMany(Rent::class);
In the beginning of calculating rents, one important concept that needs to be considered is the fact that relationships are query builders. Therefore, you could introduce additional restrictions (like rent due in the period between dates, or a certain amount or minimum payments.) And then, join these constraints in order to get your desired outcome:
$rents = Tenant::find(10)->rent()->where('payment', '>', 500)->first();
As with the first one similar to the first, you can substitute both foreign and local keys with the following logic:
return $this->hasMany(Rent::class, "foreign_key");
return $this->hasMany(Rent::class, "foreign_key", "local_key");
We now have all of the details on rent that is to be paid out by tenant but what happens when we've got a sum for the rent but want to know is the source of a renter's earnings is? It is possible to use belongingsToproperty. isToproperty:
belongsTo(Tenant::class);
The property is available for leasing. Home is available
$tenant = Rent::find(1)->tenant;
When we employ isTo in conjunction with the isTo method, we're capable of further overwriting each key to make local and foreign keys exactly like it was in the past.
It's just one of the multiple connections
Since our Tenant model was created to integrate with a variety of Rent models, it's important that you have access to the most recent and accurate versions of the model that describes the relationship.
The most efficient way to accomplish this is to combine the haveOne ofMany and ofMany strategies:
public function latestRent() return $this->hasOne(Rent::class)->latestOfMany(); public function oldestRent() return $this->hasOne(Rent::class)->oldestOfMany();
In standard procedure, we're provided with relevant information regarding the key that is used as the principal. Data is then taken care of. However, you can create the filter you want with the. ofMany method.
return $this->hasOne(Rent::class)->ofMany('price', 'min');
HasOneThrough and HasManyThrough Relationships
It is evident that the traversalmethods indicate that the models we use can navigate through different models to form an alliance with the original model. In this instance, for instance, we can link the rent directly to the Landlord. It is however required to pass the rent through the Tenant prior to it gets to the landlord.
Table keys are necessary to achieve this style of design.
rent id - integer name - string value - double tenants id - integer name - string rent_id - integer landlord id - integer name - string tenant_id - integer
If we've imagined the table we'd like to see and feel, we can design the following models:
hasOneThrough(Landlord::class, Tenant::class);
The main reason for using the hasOneThrough technique is the main motive for employing this technique is to gain access to the model you'd like to have access to. The third concern is the model which you'll be traversing through.
Like in the past, you are able to alter the keys for both foreign and local. Since we have now two keys, it is possible to duplicate the following sequence:
public function rentLandlord() return $this->hasOneThrough( Landlord::class, Tenant::class, "rent_id", // Foreign key on the tenant table "tenant_id", // Foreign key on the landlord table "id", // Local key on the tenant class "id" // Local key on the tenant table );
In most cases, you'll find that you can use the "Has Numerous Through" relation within Laravel Eloquent can be useful for those trying to gain access to data in difficult-to-access tables by using the intermediary table. Consider the following example that contains three tables.
- The nation
- users
- Games
Every country offers its own unique variety of players. Every User participates with an array of Games. The goal of our site is to find every Games with a link to the nation of specific games by using the table of Users.
Tables are described in this way:
country id - integer name - string user id - integer country_id - integer name - string games id - integer user_id - integer title - string
Then, you will need to build an elegant representation of each table that you have:
hasMany(User::class); public function games() return $this->hasManyThrough(Games::class, User::class);
belongsTo(Country::class); public function posts() return $this->hasMany(Post::class);
belongsTo(User::class);
In the next step, we'll be able apply the game()method of the Country model in order be able to access any game, since we've discovered there is an "Has Many Through" relationship between Country and Game based on the User model.
games;
Many-to-Many Relationship
The link between a huge number of people could be complex. One good example is someone who has several roles. It is also possible of assigning a position to several employees. That is why there exists the multiple-to-many relation.
To be able for the process to go smoothly to achieve this, we need to incorporate employees, roles, and rolestables.
The table structure of a database is as follows:
employees id - integer name - string roles id - integer name - string role_employees user_id - integer role_id - integer
Based on the design of the table in relation and the form of the table in relation, it's easy to establish the form of our employee model as a component of the belongToMany model. model.
belongsToMany(Role::class);
Once we've defined the role, we are able to gain access to all the roles that employees play and then filter them by:
$employee = Employee::find(1); $employee->roles->forEach(function($role) // ); // OR $employee = Employee::find(1)->roles()->orderBy('name')->where('name', 'admin')->get();
As with other techniques that we have used, we can substitute local and foreign keys by using the belongsToMany. belongsToMany technique.
To determine the inverted relation of belongsToMany it is important to define the inverted relationship. You can use precisely the same process by using the child approach with parents acting as the argument.
belongsToMany(Employee::class);
The reason for The Intermediate Table
You may have noticed that, when using the many-tos connection however the need to utilize an intermediary table. In this instance we're using the employee_job_employees table.
The pivot table default contains only ID attributes. If you want to include other attributes, we'll need to include them by following the steps:
return $this->belongsToMany(Employee::class)->withPivot("active", "created_at");
If we would like to decrease the time axis, it's feasible to achieve this:
return $this->belongsToMany(Employee::class)->withTimestamps();
Something to bear in mind is it is possible to alter the term "pivot" according to the needs of our clients. We prefer it to be a match for the needs of our clients to suit our personal preferences.
return $this->belongsToMany(Employee::class)->as('subscription')->withPivot("active", "created_by");
return $this->belongsToMany(Employee::class)->wherePivot('promoted', 1); return $this->belongsToMany(Employee::class)->wherePivotIn('level', [1, 2]); return $this->belongsToMany(Employee::class)->wherePivotNotIn('level', [2, 3]); return $this->belongsToMany(Employee::class)->wherePivotBetween('posted_at', ['2023-01-01 00:00:00', '2023-01-02 00:00:00']); return $this->belongsToMany(Employee::class)->wherePivotNull('expired_at'); return $this->belongsToMany(Employee::class)->wherePivotNotNull('posted_at');
The second amazing thing is the capability to place an order via pivots.
return $this->belongsToMany(Employee::class) ->where('promoted', true) ->orderByPivot('hired_at', 'desc');
Polymorphic Relationships
The term Polymorphic is derived from Greek that means "many types of." The app's layout might include different types of connections. The app could have more than one connection. Imagine we're creating an application which includes blog entries and videos, as well as polls. Users can leave comments about each. This means that it's possible to comment about any one of them. The way to comment can be found in blogs, Videos, as well as polls situations.
The One To One Polymorphism
This type of relationship is akin to a typical one-to-1 relationship. There is one difference: the child model can be connected to different kinds of models sharing the identical relationship.
Consider, as an example, one instance of this model. Tenantand Landlord model. There could be some interpolymorphic relationship to WaterBill. WaterBill models.
The structure of tables could look like:
tenants id - integer name - string landlords id - integer name - string waterbills id - integer amount - double waterbillable_id waterbillable_type
The waterbillable_id column can be used for identification purposes for the tenant, the landlordor an ownerwhile the column for waterbillable_type is the name of the class used in the model's parent. The type column is used to make it clear, to identify the model which is creating.
The definition model of this relationship type could comprise:
morphTo(); class Tenant extends Model public function waterBill() return $this->morphOne(WaterBill::class, 'billable'); class Landlord extends Model public function waterBill() return $this->morphOne(WaterBill::class, 'billable');
After everything has been set up and functioning properly, we'll get access to information about models like the Tenant as well as Landlord models.
waterBill; $landlord = Landlord::find(1)->waterBill;
This is known as the Polymorphic One to Many
Like the one-to-many model. There is a major difference in the way that children models can be a part of a variety of models through the creation of an association.
If you're on a social media site like Facebook you are able to leave comments on your posts, videos as well as polls in real-time. Utilizing a polymorphic model design that is used to make tables from various sources and tables, you can create an table that could be used to save comments tables for making comments in various kinds of categories. The design of the table can be described according to:
posts id - integer title - string body - text videos id - integer title - string url - string polls id - integer title - string comments id - integer body - text commentable_id - integer commentable_type - string
The commentable_id is the number of the record. The commentable_type is the type of class, and anybody who's competent in speaking will be able to recognize what they're trying to find. Its design is similar to polymorphic ones that are many
morphTo(); class Poll extends Model public function comments() return $this->morphMany(Comment::class, 'commentable'); class Live extends Model public function comments() return $this->morphMany(Comments::class, 'commentable');
For the purpose of finding comments that have been posted on the stream live, we need to employ the Find method. It makes utilization of ID. now we have access to the class that allows comments:
comments as $comment) // OR Live::find(1)->comments()->each(function($comment) // ); Live::find(1)->comments()->map(function($comment) // ); Live::find(1)->comments()->filter(function($comment) // ); // etc.
If we're the owner of the comments, and want to know who posted them Comments can be used by the method of Commentable:
Type that allows the use of comments on Post, Video, or Poll Live
One that is polymorphic of many
There's a wide range of applications that have a large number of users, they require an efficient and quick way to communicate with models, and with models. It is possible that the requirement arises as a result of a user's initial or final posting that could be accomplished using a mix of ofMany, MorphOne as well as ofMany methods.
morphOne(Post::class, 'postable')->latestOfMany(); public function oldestPost() return $this->morphOne(Post::class, 'postable')->oldestOfMany();
The two methods of the latestOfMany and olderOfManyare using the latest or the oldest model. It is built on the model's initial primary and must be able to sort.
There are times when it is not necessary to classify the content using an ID. Maybe we've changed the publication date for some posts, however it is important to categorize them in accordance with the order in which they were published rather than according to an ID number.
This is accomplished by transferring two parameters into ofMany. ofMany is one of the techniques that could help with this. First, we must choose the primary that is to be chosen. The next element is the way of sorting:
morphOne(Post::class, "postable")->ofMany("published_at", "max");
In this way, you'll be able to create high-level connections through connecting to them! Take this as an example. There is a need to gather an inventory of every article about news that are published in chronological order in relation to when they first came out. This is a problem should there are two articles that have the same date of publication. Also, the articles are that are scheduled for publication in the near future.
This way, you can pass the order we want for the filter to be used using ofMany. ofMany method. By doing this it will arrange articles according to the date they were published at. If they're alike, the publishing order is determined using ID. Additionally, we could add the query function to ofMany within of the ofMany procedure to stop the publication of any articles that requires publication!
hasOne(Post::class)->ofMany([ 'published_at' => 'max', 'id' => 'max', ], function ($query) $query->where('published_at', '
Multiplemorphic polymorphic many to Many
The polymorphic model applied to many-to-many objects can be more complicated than the normal one. Most commonly, it is where tags can be added to a variety of objects within your app. For instance, in the case of TikTok there are tags which could be used to create videos, shorts and stories along with other forms of media.
The polymorphic many-to-many feature allows us to make tables of tags that can be utilized for video or short films or stories.
The table structure is easy to comprehend:
videos id - integer description - string stories id - integer description - string taggables tag_id - integer taggable_id - integer taggable_type - string
After the tables are complete, when the tables are completed and ready, we can build models using the MorphToMany method. It accepts names for the class of the model, as well as names for relationships':
morphToMany(Tag::class, 'taggable');
And with this it is possible to construct an inverted relation. Each model which has children can be defined by this phrase as"morphedByMany". morphedByMany technique.
morphedByMany(Story::class, 'taggable'); public function videos() return $this->morphedByMany(Video::class, 'taggable');
If we're offered tags that we'd want to apply for we can locate all videos and stories associated with the tag!
stories; $videos = $tag->stories;
Improve Eloquent in order to speed it up
Laravel is an extremely efficient caching software that can be used with multiple backends like Redis, Memcached in addition to caching that is built upon the file. By caching the result of the query, you are able to reduce the volume of queries that database servers run and also increase the effectiveness of your application and efficient.
You can also use the query builder to construct more intricate queries. This can improve the speed of your site.
Summary
Eloquent relation are a important feature of Laravel that lets programmers efficiently handle data linked to each other. From one-to-1 connections to many-to-1 ones Eloquent is a simple and easy syntax for defining and analyze the relation.
This article was originally posted on this site
The original article appeared on this website
This post first came up here. this site
Article was first seen on here